-
Recall from Lesson A2 that an object is constructed as an instance of a particular class. The object is most often referenced using an identifier. The identifier is a variable that stores the reference to the object. This identifier is called an object reference. Now that we are working with a simple class, String
, it is a good time to discuss object references.
-
Whenever the new operator is used, a new object is created. Each time an object is created, there is a reference to where it is stored in memory. The reference can be saved in a variable. The reference is used to find the object.
- It is possible to store a new object reference in a variable. For example:
String str;
str = new String("first string");
System.out.println(str);
str = new String("second string");
System.out.println(str);
Run Output:
first string
second string
In the example above, the variable str
is used to store a reference to the String
, “first string”. In the second part of the example a reference to the String
, “second string” is stored in the variable str
. If another reference is saved in the variable, it replaces the previous reference (see diagram below).
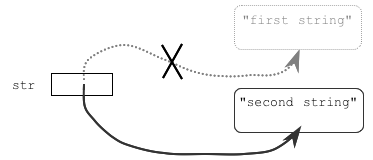
-
If a reference to an object is no longer being used then there is no way to find it, and it becomes "garbage." The word "garbage" is the correct term from computer science to use for objects that have no references. This is a common situation when new objects are created and old ones become unneeded during the execution of a program. While a program is running, a part of the Java system called the "garbage collector" reclaims each lost object (the "garbage") so that the memory is available again. In the above example, the String
object “first string” becomes garbage.
- Multiple objects of the same class can be maintained by creating unique reference variables for each object.
String strA; // reference to the first object
String strB; // reference to the second object
// create the first object and save its reference
strA = new String("first string");
// print data referenced by the first object.
System.out.println(strA);
// create the second object and save its reference
strB = new String("second string");
// print data referenced by the second object.
System.out.println(strB);
// print data referenced by the first object.
System.out.println(strA);
Run Output:
first string
second string
first string
This program has two reference variables, strA
and strB
. It creates two objects and places each reference in one of the variables. Since each object has its own reference variable, no reference is lost, and no object becomes garbage (until the program has finished running).
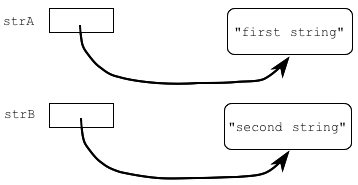
- Different reference variables that refer to the same object are called aliases. In effect, there are two names for the same object. For example:
String strA; // reference to the object
String strB; // another reference to the object
// Create the only object and save its
// reference in strA
strA = new String("only one string");
System.out.println(strA);
strB = strA; // copy the reference to strB.
System.out.println(strB);
Run Output:
only one string
only one string
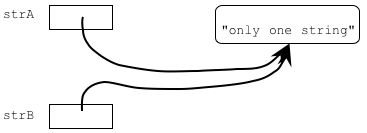
When this program runs, only one object is created (by new
). Information about how to find the object is put into strA
. The assignment operator in the statement
strB = strA; // copy the reference to strB
copies the information that is in strA
to strB
. It does not make a copy of the object.